Dünya çapında binlerce pazarlamacı tarafından güvenilir
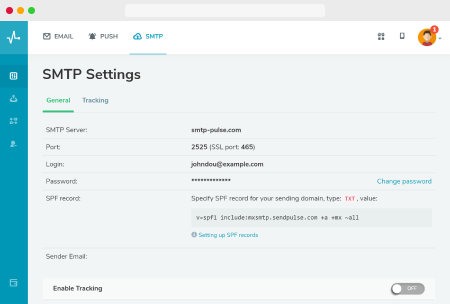
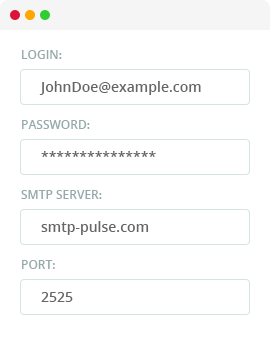
SMTP
İşlemsel e-postalar, bir abonenin işlemlerine yanıt olarak gönderilen otomatik iletilerdir. Abonelik veya rezervasyon onayı, sipariş güncellemeleri, bildirimler - bunlar işlem e-postalarının örnekleridir.
SMTP ile işlemsel e-posta göndermek için, bir sunucu adresi, bağlantı noktası, giriş ve SendPulse hesabınızın şifresini uygulamanıza girin.
<?php
use Sendpulse\RestApi\ApiClient;
use Sendpulse\RestApi\Storage\FileStorage;
$smtpSendMailResult = (new ApiClient('MY_API_ID', 'MY_API_SECRET', new FileStorage()))->post('smtp/emails', [
'email' => [
'html' => base64_encode('<p>Hello!</p>'),
'text' => 'text',
'subject' => 'Mail subject',
'from' => ['name' => 'API package test', 'email' => 'from@test.com'],
'to' => [['name' => 'to', 'email' => 'to@test.com']],
'attachments_binary' => [
'attach_image.jpg' => base64_encode(file_get_contents('../storage/attach_image.jpg'))
]
]]);
var_dump($smtpSendMailResult);
?>
sample.php hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-php
# SendPulse's Ruby Library: https://github.com/sendpulse/sendpulse-rest-api-ruby
require './api/sendpulse_api'
sendpulse_api = SendpulseApi.new(API_CLIENT_ID, API_CLIENT_SECRET, API_PROTOCOL, API_TOKEN)
email = {
html: '<p>Your email content goes here<p>',
text: 'Your email text version goes here',
subject: 'Testing SendPulse API',
from: { name: 'Your Sender Name', email: 'your-sender-email@gmail.com' },
to: [
{
name: "Subscriber's name",
email: 'subscriber@gmail.com'
}
]
}
sendpulse_api.smtp_send_mail(email)
sample.rb hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-ruby
# SendPulse's Python Library: https://github.com/sendpulse/sendpulse-rest-api-python
from pysendpulse import PySendPulse
if __name__ == "__main__":
TOKEN_STORAGE = 'memcached'
SPApiProxy = PySendPulse(REST_API_ID, REST_API_SECRET, TOKEN_STORAGE)
email = {
'subject': 'This is the test task from REST API',
'html': '<p>This is a test task from https://sendpulse.com/api REST API!<p>',
'text': 'This is a test task from https://sendpulse.com/api REST API!',
'from': {'name': 'John Doe', 'email': 'john.doe@example.com'},
'to': [
{'name': 'Jane Roe', 'email': 'jane.roe@example.com'}
]
}
SPApiProxy.smtp_send_mail(email)
sample.py hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-python
// SendPulse's Java Library https://github.com/sendpulse/sendpulse-rest-api-java
import com.sendpulse.restapi.Sendpulse;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
public class Example {
public static void main(String[] args) {
Sendpulse sendpulse = new Sendpulse(API_CLIENT_ID, API_CLIENT_SECRET);
Map<String, Object> from = new HashMap<String, Object>();
from.put("name", "Your Sender Name");
from.put("email", "your-sender-email@gmail.com");
ArrayList<Map> to = new ArrayList<Map>();
Map<String, Object> elementto = new HashMap<String, Object>();
elementto.put("name", "Subscriber's name");
elementto.put("email", "subscriber@gmail.com");
to.add(elementto);
Map<String, Object> emaildata = new HashMap<String, Object>();
emaildata.put("html","Your email content goes here");
emaildata.put("text","Your email text version goes here");
emaildata.put("subject","Testing SendPulse API");
emaildata.put("from",from);
emaildata.put("to",to);
Map<String, Object> result = (Map<String, Object>) sendpulse.smtpSendMail(emaildata);
System.out.println("Result: " + result);
}
}
sample.java hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-java
// SendPulse's Node.JS Library: https://github.com/sendpulse/sendpulse-rest-api-node.js
var sendpulse = require("./api/sendpulse.js");
sendpulse.init(API_USER_ID, API_SECRET, TOKEN_STORAGE);
var email = {
"html" : "<p>Your email content goes here</p>",
"text" : "Your email text version goes here",
"subject" : "Testing SendPulse API",
"from" : {
"name" : "Your Sender Name",
"email" : "your-sender-email@gmail.com"
},
"to" : [ {
"name" : "Subscriber's name",
"email" : "subscriber@gmail.com"
} ]
};
var answerGetter = function answerGetter(data){
console.log(data);
}
sendpulse.smtpSendMail(answerGetter, email);
sample.js hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-//www.spcdn.org/node.js
#import "Sendpulse.h" // SendPulse's Obj-C Library https://github.com/sendpulse/sendpulse-rest-api-objective-c
- (void)viewDidLoad {
[super viewDidLoad];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(doSomethingWithTheData:) name:@"SendPulseNotification" object:nil];
Sendpulse* sendpulse = [[Sendpulse alloc] initWithUserIdandSecret:userId :secret];
NSDictionary *from = [NSDictionary dictionaryWithObjectsAndKeys:@"Your Sender Name", @"name", @"your-sender-email@gmail.com", @"email", nil];
NSMutableArray* to = [[NSMutableArray alloc] initWithObjects:[NSDictionary dictionaryWithObjectsAndKeys:@"Subscriber's name", @"name", @"subscriber@gmail.com", @"email", nil], nil];
NSMutableDictionary *emaildata = [NSMutableDictionary dictionaryWithObjectsAndKeys:@"<b>Your email content goes here</b>", @"html", @"Your email text version goes here", @"text",@"Testing SendPulse API",@"subject",from,@"from",to,@"to", nil];
[sendpulse smtpSendMail:emaildata];
}
- (void)doSomethingWithTheData:(NSNotification *)notification {
NSMutableDictionary * result = [[notification userInfo] objectForKey:@"SendPulseData"];
NSLog(@"Result: %@", result);
}
sample.m hosted with ❤ by
github.com/sendpulse/sendpulse-rest-api-objective-cSMTP API'sı
SMTP API, abonelerinize web sitenizden, CRM'den veya diğer web uygulamalarından işlem e-postaları göndermenizi sağlar.
E-posta etkinliği raporları
E-posta pazarlama performansınızı ve YG'nizi iyileştirmek için e-posta metriklerini düzenli olarak ölçün. Tüm SendPulse raporları - açık ve tıklama oranlarında, gelen kutusu yerleşimi, gönderim ücretleri ve hatalar ve spam şikayetleriyle ilgili olarak indirilebilir.
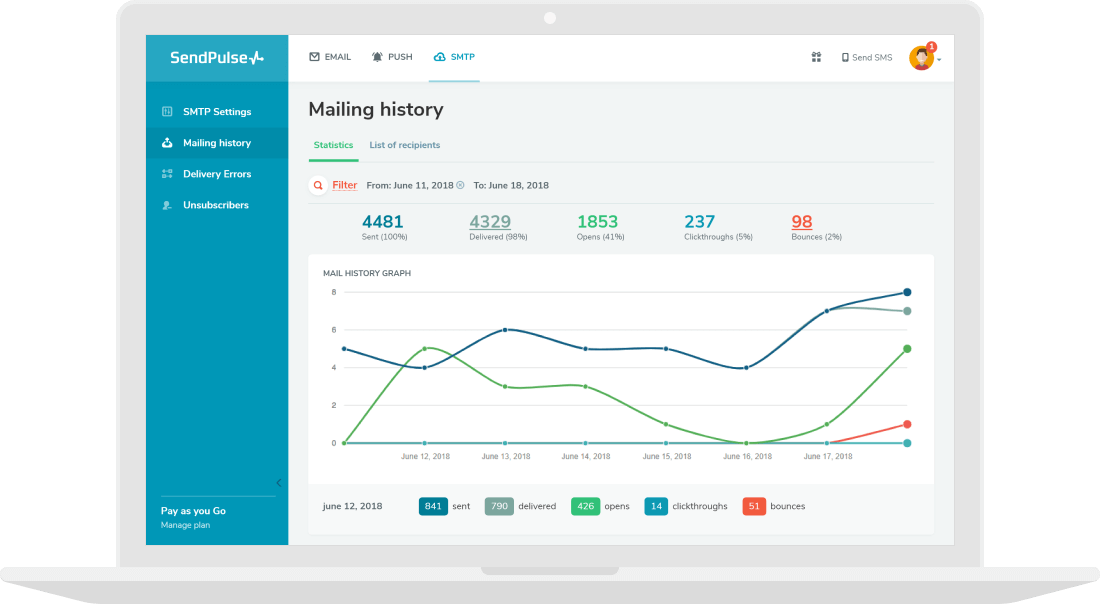
İşlem e-postaları göndermek için kullanımı kolay araçlar
Özel IP adresleri
Özel bir adres daha iyi bir gönderici itibarına katkıda bulunur ve IP'nizin kara listeye alınmasını önler.
SPF ve DKIM kimlik doğrulaması
SPF ve DKIM kayıtları, işlem e-postalarının bir spam klasörüne gitmesini engeller.
Abone olmayan kişilerin listesi
E-posta adresiniz, posta listesinde olsalar bile abone olmayan kişilere gönderilmez.
Açılma ve tıklama takibi
Ayrıntılı raporlar, toplu mail pazarlama etkinliğini değerlendirmek ve iyileştirmek için yardımcı olur.
Özel İzleme Alanı
E-posta üstbilgisinde ve altbilgisinde SendPulse'den herhangi bir şey belirtmeden işlem e-postaları gönderin.
Webhooks
Sisteminize e-posta durumuyla ilgili bilgi edinin: teslim edilen, teslim edilmemiş, açılmış ve tıklanmış.
veya